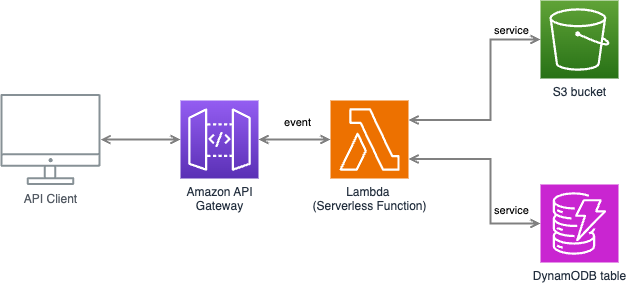
Serverless functions are transforming modern application development by eliminating the need for server management. These event-driven, scalable, and cost-efficient code units allow developers to focus on writing logic without worrying about infrastructure. In this guide, you’ll learn what serverless functions are, how they work, their benefits, and why platforms like AWS Lambda, Azure Functions, and Google Cloud Run functions are leading this space.
Contents
What Are Serverless Functions?
Serverless functions are small units of code that execute in response to specific events, such as HTTP requests, database triggers, or message queues. Note that the name ‘functions’ is not the same as functions/methods used in programming languages like C and C++. These functions can be multiple methods in multiple files working together to fulfill a use case or business logic. These functions are stateless, meaning they don’t retain any data between executions. Cloud platforms like AWS, Azure, and Google Cloud provide the runtime environment for these functions.
Why Go Serverless?
- No Server Management
Forget provisioning, scaling, or maintaining servers. The cloud provider handles all of that for you. - Cost-Effective
Pay only for the time your code runs, measured in milliseconds. No charges for idle time! - Scalable by Default
Serverless functions automatically scale up or down based on the number of incoming requests. - Quick to Deploy
Focus on the code logic without worrying about the underlying infrastructure.
How Serverless Functions Work
- Event Triggers
An event, like a user request or a database change, triggers the function. - Execution
The cloud provider spins up an environment, runs your code, and handles the result. - Scaling
If multiple events occur simultaneously, the provider creates new instances of the function as needed.
Example: AWS Lambda
Here’s a simple example of an AWS Lambda function that responds to an HTTP request:
import json def lambda_handler(event, context): # Extract the 'name' query parameter from the HTTP request name = event.get('queryStringParameters', {}).get('name', 'World') # Return a JSON response return { "statusCode": 200, "body": json.dumps({"message": f"Hello, {name}!"}), "headers": { "Content-Type": "application/json" } }
- Trigger: This function can be triggered by an API Gateway when an HTTP request is made.
- Query Parameter: It looks for a query parameter
name
(e.g.,?name=John
) in the incoming request. - Response: Returns a JSON object containing a personalized greeting.
Deploy this function using the AWS Console, and your code is live within minutes.
Common Use Cases
- Real-Time File Processing: Resize images or transcode videos on the fly.
- APIs: Build RESTful or GraphQL APIs without managing servers.
- Automation: Automate workflows, such as sending emails or updating databases.
- IoT: Handle incoming data from IoT devices with low latency.
Challenges to Keep in Mind
- Cold Starts: Initial execution might have a slight delay when the function is idle for a while.
- Stateless Nature: Requires external storage for maintaining persistent data.
- Vendor Lock-In: Migrating functions across cloud providers can be complex.
Takeaway
Serverless functions simplify development by eliminating infrastructure management, reducing costs, and offering scalability. Whether you’re a startup building your first API or an enterprise automating workflows, serverless functions provide the flexibility and efficiency you need.
What Next?
Ready to go Serverless? Start experimenting with platforms like AWS Lambda, Azure Functions, or Google Cloud Run functions to see how serverless fits into your next project.