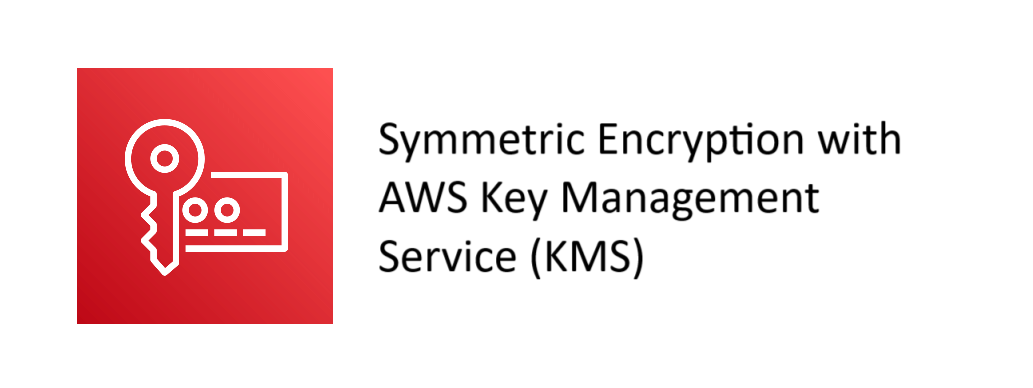
Contents
Introduction
When handling sensitive data in AWS, ensuring that data is encrypted is critical to maintaining its security. AWS Key Management Service (KMS) offers a robust way to encrypt and decrypt data using symmetric encryption keys. This article demonstrates how to use KMS to secure your data that is read from and/or written to databases like DynamoDB or RDS or passed back and forth in services like API Gateway.
Why Use AWS KMS?
AWS KMS simplifies encryption by providing the following features:
Centralized Key Management
KMS enables you to create, rotate, and manage keys from a single service. KMS supports both AWS Managed Keys and Customer Managed Keys.
Seamless Integration
Works directly with many AWS services. Data encrypted in one service can be seamlessly decrypted from another service.
Security Features
Hardware Security Modules (HSMs) offer a dedicated, tamper-resistant environment for managing encryption keys. AWS KMS integrates with HSMs to provide secure key storage and operations. Using HSM-backed keys enhances protection against physical and remote attacks, ensuring the highest level of security for your sensitive data. HSMs are particularly valuable for compliance with stringent regulatory standards.
Key Rotation
KMS provides automatic key rotation and on-demand key rotation feature to comply with industry standards and compliance requirements.
Encrypting Data using KMS
To encrypt data within the AWS environment, you must first create a key. A key can be AWS-managed or Customer-managed. AWS-managed keys are automatically created and managed by AWS when certain services are created and configured (like DynamoDB tables, RDS databases and Lambda functions). In this example, we’ll create a customer-managed key.
Boto3 Authentication
There are different ways to configure AWS authentication while using boto3 library locally. A list of such methods can be found here.
Create a Customer-Managed Key
To create a customer-managed key, follow these steps:
import boto3 kms_client = boto3.client('kms') response = kms_client.create_key( Description='Key for encrypting DynamoDB data', KeyUsage='ENCRYPT_DECRYPT', CustomerMasterKeySpec='SYMMETRIC_DEFAULT' ) key_id = response['KeyMetadata']['KeyId'] print(f"Created KMS Key with ID: {key_id}")
Go to KMS -> Customer managed keys in the AWS console, and you can verify that the key has been created.

Encrypt Data
Use the KMS encrypt
method to perform client-side encryption:
import boto3 kms_client = boto3.client('kms') dynamodb_client = boto3.client('dynamodb') # Encrypt sensitive data plaintext = b"Sensitive information" response = kms_client.encrypt( KeyId=key_id, Plaintext=plaintext ) encrypted_data = response['CiphertextBlob'] print(encrypted_data) # Store encrypted data in DynamoDB dynamodb_client.put_item( TableName='YourTableName', Item={ 'PrimaryKey': {'S': 'YourPrimaryKeyValue'}, 'EncryptedData': {'B': encrypted_data} } )
This example shows how to store encrypted data in a DynamoDB table.
Decrypt Data
To decrypt the encrypted data:
# Retrieve encrypted data from DynamoDB response = dynamodb_client.get_item( TableName='YourTableName', Key={'PrimaryKey': {'S': 'YourPrimaryKeyValue'}} ) encrypted_data = response['Item']['EncryptedData']['B'] # Decrypt the data response = kms_client.decrypt( KeyId=key_id, CiphertextBlob=encrypted_data ) decrypted_data = response['Plaintext'] print(f"Decrypted data: {decrypted_data.decode()}")
It’s not necessary to specify the Key id during decryption as KMS can automatically infer the Key Id from the Cipher metadata. However, it is always a good practice to specify the Key Id.
Key Rotation and Protection
Key Rotation
AWS KMS supports automatic key rotation. Enable rotation for your customer-managed key. KMS automatically stores previous keys so that your data encrypted with an older key is automatically decrypted with the same key even if the key changes between the encryption and decryption process. The enable_key_rotation API takes two parameters: the key id and the rotation period in days which can can be between 90 and 2560 days. If no rotation period is specified, it defaults to 365 days.
kms_client.enable_key_rotation(KeyId=key_id, RotationPeriodInDays=90) print("Key rotation enabled.")
You can verify in the Key rotation tab in the Key properties in the AWS console that automatic key rotation has been enabled.

In addition to automatic key rotation, KMS also provides on-demand key rotation that can be used during incidents when a key has been compromised. This prevents new data being encrypted with the compromised keys. Note, however, that on-demand rotation can be performed up to 10 times only on a key.

Protecting KMS Keys
Enable Key Policies
Use key policies to define fine-grained access control.
{ "Version": "2012-10-17", "Id": "key-default-1", "Statement": [ { "Sid": "Enable IAM User Permissions", "Effect": "Allow", "Principal": { "AWS": "arn:aws:iam::123456789012:root" }, "Action": "kms:*", "Resource": "*" } ] }
Audit Key Usage
Monitor key usage with AWS CloudTrail. Use AWS CloudTrail to monitor KMS key activity. CloudTrail logs all API calls made to AWS services, including KMS. By enabling CloudTrail, you can track key creation, encryption, decryption, and deletion operations. This visibility helps you detect unauthorized access and ensures compliance with security policies.
Enable Multi-Factor Authentication (MFA)
Multi-Factor Authentication (MFA) adds an additional layer of security to critical KMS operations, such as key management or deletion. By requiring a second form of authentication, you reduce the risk of unauthorized access even if an account credential is compromised. Configure MFA in your IAM policies to protect sensitive operations effectively.
Disable Keys
You can disable a key quickly in case of a security breach or a key compromise incident. Disabled keys cannot be used for encryption and decryption.
Conclusion
Using AWS KMS for symmetric encryption enhances the security of your data in AWS environment. By combining server-side encryption with client-side encryption and adhering to best practices like key rotation and protection, you can create a robust security framework for your applications. Secure your data today with AWS KMS to ensure compliance and protect against data breaches.
[…] Protect your data with Symmetric Encryption in AWS using KMS […]